Linting is a way to automatically check code for potential errors and formatting issues.
Once code standards are defined for your WordPress project, linting issues can be displayed directly in VS Code as you work and most code formatting issues can be autocorrected on save.
See How it Works
To get started, you’ll need to have Composer installed locally. This setup uses the WordPressVIPMinimum and WordPress-Extra rulesets. If you want to see a working example, check out my WooCommerce Coupon Restrictions extension on GitHub.
Set up composer.json file
Linting can be set up for a plugin, theme, or an entire WordPress site. To get started, create a composer.json file in the root of your project directory.
To use the WordPressVIPMinimum and WordPress-Extra rulesets you’ll need to install two composer packages.
composer require dealerdirect/phpcodesniffer-composer-installer --dev
composer require automattic/vipwpcs --dev
Set up phpcs.xml
Next we’ll need to define the rulesets to use and which files or directories to check. That happens in the phpcs.xml file, which also goes in the root of your project directory. See this phpcs.xml as an example.
The file contents should look something like this:
<?xml version="1.0"?>
<ruleset>
<arg name="basepath" value="."/>
<arg name="extensions" value="php"/>
<arg name="severity" value="4"/>
<arg name="tab-width" value="4"/>
<arg name="parallel" value="80"/>
<arg name="cache" value=".phpcs-cache"/>
<arg name="colors"/>
<exclude-pattern>*/.git/*</exclude-pattern>
<exclude-pattern>.github/</exclude-pattern>
<exclude-pattern>*/vendor/*</exclude-pattern>
<exclude-pattern>*/node_modules/*</exclude-pattern>
<exclude-pattern>*/tests/*</exclude-pattern>
<exclude-pattern>*/bin/*</exclude-pattern>
<config name="testVersion" value="7.4"/>
<config name="php_version" value="70407"/>
<!-- Ignore warnings, show progress of the run and show sniff names -->
<arg value="nps"/>
<!-- Directories to be checked -->
<file>.</file>
<!-- WordPress -->
<rule ref="WordPressVIPMinimum"/>
<rule ref="WordPress-Extra"/>
<rule ref="PSR2.Methods.FunctionClosingBrace"/>
</ruleset>
Run Linting and Fixing via Command Line
To see if everything is set up correctly, you can the checks via the command line.
To lint your codebase, run:
./vendor/bin/phpcs
If everything is working correctly, you should see an output like this:
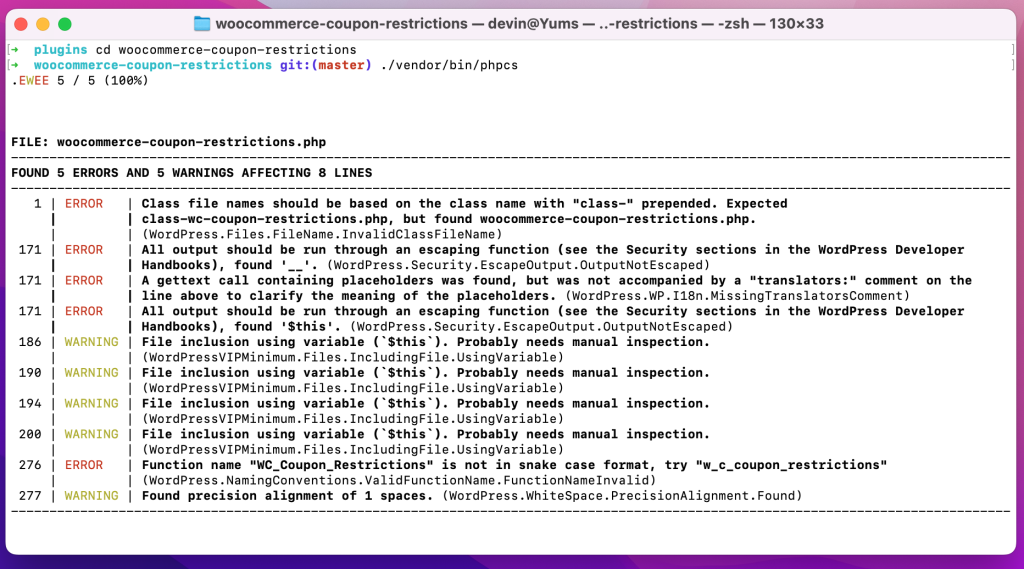
To autofix linting issues (that can be corrected automatically, run:
./vendor/bin/phpcbf
Setup for VS Code
There’s a number of different VS Code extensions that integrate with PHPCS, but setup that has worked best for me is PHP Intelephense with PHP Sniffer & Beautifier. I also highly recommend setting an .editorconfig file for you project, and installing EditorConfig for VS Code to define you indent style and sizes.
Once you have these extensions installed, you should see linting issues highlighted directly in VS Code. Formatting and spacing issues can also be autoformatted and fixed on save.